Build a React Native Chat App: A Step-by-Step Guide (2025)
Building a react native chat app using the best-in-class React Native chat SDK library is now simple & quick. Want to know how? Let’s get started.
In this tutorial, we will cover every detailed guide on how to build a React Native real-time chat app? with enriched features of sharing videos, image uploads, read state, reactions, threads, and much more.
Let’s begin!
Table of Contents
Why Choose React Native?
React Native is a JavaScript framework meant for writing real, natively rendering mobile applications like iOS and Android. It gives space for the developers to share the same codes across multiple platforms to build their react native messaging app. Furthermore, it has the potential to expand to future platforms as well.
Some of the social media platforms like Facebook, TaskRabbit, and Palantir, are utilizing React Native in production for better user-facing applications.
How to Create A React Native Chat App for Web & Mobile
To make this tutorial section much easier for you to understand, let’s get into the technical part of this chat app React native development for web applications.
Step-by-Step Guide to Create Your React Native Chat App Tutorial
Follow these essential steps to create a React Native messaging app using MirrorFly React Native API:
Step 1: Build a Sample App
- Installing Modules
- License Key
- Run the Application
Step 2: Integrate Sample App into an Existing Application with the below set of processes
- Initialize SDK
- Register
- Login
- Update User’s Profile
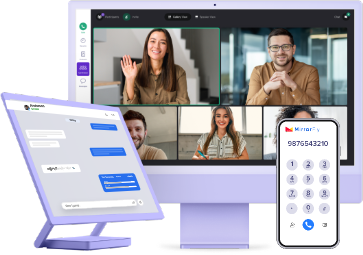
- Complete Source Code
- Lifetime Data Ownership
- Deploy on Own Server
Build a React Native Chat App for Web
Whether you want to develop a new React native chat app or integrate the chat features into the existing web application, all you need is our sample app with a sandbox SDK. Yes! our chat comes with a fully functional UI component that makes the entire development process more simple to add chat to your web.
So with all the backend coding parts, Let’s get into the detailed description of these above-mentioned steps
Before you Get Started
- Sign up for a MirrorFly account.
- Install the following dependencies
- Node – 14.20.0
- npm – 6.14.10
- react-native – 0.69.12
- Install the npm package dependencies
{
"@react-native-async-storage/async-storage": "^1.17.7",//Any version
"react-native-get-random-values": "1.7.1",//must use version >=1.7.1
"react-native-document-picker" :"8.1.1",// must use version >=8.1.1
'realm': "^10.8.0",
'react-native-fs': "^2.18.0",
'@react-native-community/netinfo': "^8.0.0",
'moment': "2.29.4",
'rn-fetch-blob': "^0.12.0",
'react-native-compressor': "1.6.1",
'react-native-convert-ph-asset': "^1.0.3",
'react-native-mov-to-mp4' :"^0.2.2"
}
Step 1: Integrate Chat SDK
- Check for the latest update of your packages.json files.
- If your iOS app is having issues, check if there are any duplicated items in your package files.
- Go to the dist folder and copy all the extracted files.
- Make a new folder within your project and then move all the copied files into that folder.
- Create a file called “SDK.js” to export the SDK objects. You can use the provided code snippets to import the SDKs.
import './index';
const SDK = window.SDK;
export default SDK;
Step 2: Initialize The Chat SDK
To initialize the Chat SDK, you’ll need specific data that reflects changes in the app’s connection status. Here’s how to do it:
- First, place the license key in the “licensekey” parameter.
- Then, use the method provided below to pass this data to the SDK for further processing
const initializeObj = {
apiBaseUrl: `API_URL`,
licenseKey: `LICENSE_KEY`,
isTrialLicenseKey: `TRIAL_MODE`,
callbackListeners: {},
};
await SDK.initializeSDK(initializeObj);
Sandbox Details
function connectionListener(response) {
if (response.status === "CONNECTED") {
console.log("Connection Established");
} else if (response.status === "DISCONNECTED") {
console.log("Disconnected");
}
}
const initializeObj = {
apiBaseUrl: "https://api-preprod-sandbox.mirrorfly.com/api/v1",
licenseKey: "XXXXXXXXXXXXXXXXX",
isTrialLicenseKey: true,
callbackListeners: {
connectionListener
},
};
await SDK.initializeSDK(initializeObj);
Example Response
{
"statusCode": 200,
"message": "Success"
}
Step 3: Register A New User
Here’s how to register a new user:
- Utilize the method provided below to register a new user.
- After registering, you’ll receive a username and password. You can use these credentials to connect to the server using the “connect” method.
await SDK.register(`USER_IDENTIFIER`);
Sample Response
{
statusCode: 200,
message: "Success",
data: {
username: "123456789",
password: "987654321"
}
}
Step 4: Connect To MirrorFly Server
Follow the below steps to connect to the MirrorFly server:
- Use the credentials you obtained during registration to establish a connection with the server.
- After successfully creating the connection, you’ll either receive an approval message with a ‘statusCode of 200’ or an error if there’s an issue.
- You can also monitor the connection status through the connectionListener callback function.
- If there’s an error during the connection process, you’ll receive an error message through a callback.
await SDK.connect(`USERNAME`, `PASSWORD`);
Sample Response
{
message: "Login Success",
statusCode: 200
}
Step 5: Send A Message
To send a message to another user, simply use the following method:
await SDK.sendTextMessage(`TO_USER_JID`, `MESSAGE_BODY`,`MESSAGE_ID`,`REPLY_TO`);
Sample Response
{
"message": "",// String - Success/Error Message
"statusCode": "" // Number - status code
}
Step 6: Receive A Message
To receive a message from another user, you should set up the “messageListener” function. This function gets activated every time you receive a new message or a related event in one-to-one or group chat. Here’s how to initialize the SDK and add this callback method during the process.
function messageListener(response) {
console.log("Message Listener", response);
}
Once the entire process of registration and sync gets completed, you can refer to adding 1:1 and group chat features.
Register
To begin with, there is a need to create a new user account
If you get the autoLogin as true, then this will enable the user to log in with XMPP after successful registration.
Note – If the sandbox mode is set to true, then the users will register into the sandbox mode at the startup itself. |
await SDK.register(`USER_IDENTIFIER`, `AUTO_LOGIN`);
Sample Responses -
If autoLogin is true
{
statusCode: 200,
message: "Login Success"
}
if autoLogin is false
{
statusCode: 200,
message: "Success",
data: {
username: "123456789",
password: "987654321"
}
}
Now use the credentials that have been received from the Register response, log in using SDK
await SDK.login(`USERNAME`, `PASSWORD`);
Check for further Request and Responses
Example Request
Image as a URL
await SDK.setUserProfile(
"Name",
"https://domain/path/image.png",
"status",
"1111111111",
"email@domain",
);
Image as a FILE
await SDK.setUserProfile("Name", FILE, "status", "1111111111", "email@domain");
When you want to sync the contacts with the License Key, use can use the Sync Contact Method
await SDK.syncContacts(`USER_IDENTIFIER`);
Once the entire process of registration and sync gets completed, you can refer to other profiles using one-to-one chat, and group chat methods.
Well, after having better clarity with the importance of implementation of React Native in your chat app. Let’s get some ideas about the market launch strategy.
Recommended Reading
8 High-end Benefits of A React Native Chat App
- Highly Compatible across the Platforms – specifies that the application that has been coded for Android can also be shared for iOS, which indeed saves time as well as cost.
- Robust Community – this provides access to user support and many other user-created tools.
- Cost Effective and Time Saving – as you can create a single app and use it for both iOS as well as Android.
- Top Reloading Feature – works across all mobile platforms with ease.
- OTA (Over The Air) – an innovative update that enables developers to let their users create and administer recent updates.
- Highly Flexible – This allows any number of developers to work on the app project at once without any interruption enabling them to add any number of features.
- Open Source Framework – this attribute provides the developers with easy accessibility to migrate from one platform to another.
Key Points to Be Considered Before Launching a React Native Chat App!
The main target of every application is to reach the market, but this is not an easy journey. Let’s have a look at some of the key points that every React native chat app has to be considered before making market launch
- Chat Performance & Reliability: Once the chat app using react native SDK is developed, it is time for it to be tested for its performance and reliability. The performance issues will be detected upon real-time experience with perceptible latency, undelivered messages, or any app crash during the course.
- Privacy & Security Protocols: Legal issues like Terms of use and privacy policy are considered to be major issues as they need to be very accurate and must be prepared under the surveillance of a lawyer before launching through the play store.
However, there are many other factors too but above mentioned are some major points for better understanding. Now, let’s see with MirrorFly is the one for this entire React native chat app development.
Why Choose MirrorFly SDK to Build Your React Native Chat App?
When it comes to real-time communication API & SDK providers, you can find several out there – among which, choosing the best one is a big deal. But still, you can have them identified with certain key points like market experience, features, pricing, and so on.
Let’s talk about such one – The “MirrorFly,” one among the top chat API and SDK provider who have marked their market existence for more than 10 year with over 40+ worldwide satisfied customers. Well, let’s get to know more about in detail with some of the highlights
- Real-Time Communication Platform – MirroFly works with multiple modes of communication that involve messaging, voice and video. It is a highly secure solution with over 150+ chat features that fit into the demands of all kinds of businesses.
- High-Scalability – Enables to scale up to 1 billion + concurrent users around the world anytime with high-end security protocols of enterprise-grade.
- High-end Security – It provides end-to-end security over the conversations among the users globally across multiple channels with SSL security, AES 256 encryption, multi-layer protection, etc.
- Hosting Option – You can avail of both on-premises and on-cloud hosting infrastructure, wherein you can have your data stored in your cloud or else in our cloud/premises as per your business requirements with guaranteed security.
- 300+ Proficient Developers – Highly professional with perfection when it comes to an app’s delivery. MirrorFly ensures you have on-time delivery on your projects with 24/7 developers’ assistance till deployment.
- White Label Solution – MirrorFly offers ready-to-use react native chat APIs and SDKs that support you to build your white label chat app with your company’s name, logo, color and custom features. This can be easily published into any play store like Google Play, etc.
- Pricing Model – Since it is available with both SaaS and Saap-based models, you can find both pricing models here i.e., Self-managed chat solution (one-time license cost) and Cloud (pay-as-you-go) pricing model as per your business requirement.
Final Words!
I hope this chat app tutorial would have given you a better idea as to how we built a multi-tenant React Native chat app using the React Native SDKs and component library and of course, with details on how to integrate them into any pre-build device with simple changes in codes.
Well, after all this session if you feel this tutorial is an end for searching for the best mobile app development company, then feel free to contact us for further guidance. By the way, you can also check our other tutorials, for Android and iOS for better exposure.
All the Best towards the Journey to Your Chat App. Thank You!
Get Started with MirrorFly’s React Native Chat API
Drive 1+ billions of conversations on your apps with highly secure 250+ real-time Communication Features.
Contact Sales200+ Happy Clients
Topic-based Chat
Multi-tenancy Support
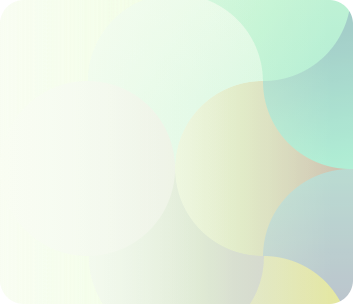
Frequently Asked Questions (FAQs)
Does WhatsApp use React Native?
No, WhatsApp doesn’t use React Native in its UI components. WhatsApp is being developed natively using platform-specific applications such as Objective-C and Swift for iOS and Kotlin and Java for Android. But as technology grows, new frameworks can be adopted by brands depending on their project requirements and programming features.
How do I make a native React chat app for free?
To build your own React Native chat app for free, you can hire a team of developers or follow the steps to create your own React Native chat app for free. But there are other options too; you can go through a list of free chat SDK providers like MirrorFly, Getstream, Cometchat, etc., and easily integrate them into your preferred platform.
How do I create a group chat in React Native?
To create a group chat in React Native, you can follow these simple steps:
Step 1: Set up the React Native project.
Step 2: Design the user interface
Step 3: Implement navigation
Step 4: Connect to the backend
Step 5: Fetch group chat data.
Step 6: Create or join a group chat.
Step 7: Display or send group chat messages.
Can I use Firebase with React Native?
Yes, you can use Firebase with React Native. Firebase’s wide portfolio of cloud-based services can be easily integrated into your React Native applications. It offers features such as a real-time database, authentication, cloud storage, messaging, and more, which can simplify the development process.
Is React a native open-source framework?
Yes, React Native is an open-source framework initially developed and released by Facebook in 2015. As an open-source framework, React Native allows developers to migrate from one platform to another. The source code of React Native is hosted on Github, which allows developers the freedom to use, modify, and distribute the code for personal and commercial needs.
Which famous brands use React Native?
Facebook, Instagram, Airbnb, Tesla, Bloomberg, Walmart, etc. are some of the popular brands that have adopted the React Native framework for their mobile applications UI components. The adoption of React Native by these popular brands proves the framework’s creativity, performance, and ability to deliver cross-platform mobile experiences proficiently.
Related Articles
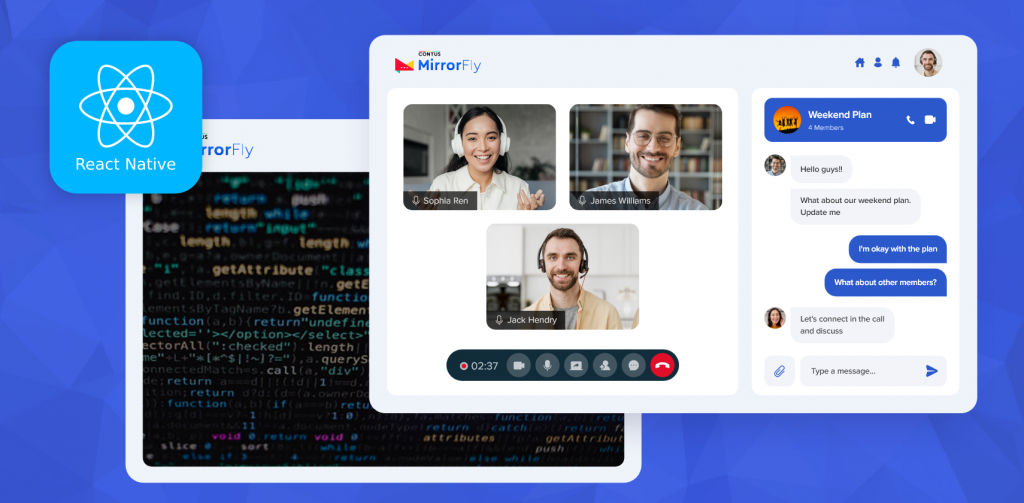
Building a react native chat app using the best-in-class React Native chat SDK library is now simple & quick. Want to know how? Let’s get started.
In this tutorial, we will cover every detailed guide on how to build a React Native real-time chat app? with enriched features of sharing videos, image uploads, read state, reactions, threads, and much more.
Let’s begin!
Table of Contents
Why Choose React Native?
React Native is a JavaScript framework meant for writing real, natively rendering mobile applications like iOS and Android. It gives space for the developers to share the same codes across multiple platforms to build their react native messaging app. Furthermore, it has the potential to expand to future platforms as well.
Some of the social media platforms like Facebook, TaskRabbit, and Palantir, are utilizing React Native in production for better user-facing applications.
How to Create A React Native Chat App for Web & Mobile
To make this tutorial section much easier for you to understand, let’s get into the technical part of this chat app React native development for web applications.
Step-by-Step Guide to Create Your React Native Chat App Tutorial
Follow these essential steps to create a React Native messaging app using MirrorFly React Native API:
Step 1: Build a Sample App
- Installing Modules
- License Key
- Run the Application
Step 2: Integrate Sample App into an Existing Application with the below set of processes
- Initialize SDK
- Register
- Login
- Update User’s Profile
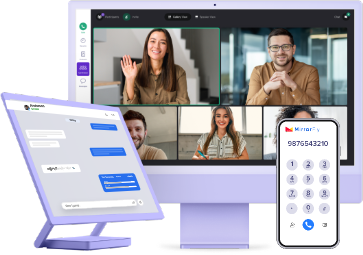
- Complete Source Code
- Lifetime Data Ownership
- Deploy on Own Server
Build a React Native Chat App for Web
Whether you want to develop a new React native chat app or integrate the chat features into the existing web application, all you need is our sample app with a sandbox SDK. Yes! our chat comes with a fully functional UI component that makes the entire development process more simple to add chat to your web.
So with all the backend coding parts, Let’s get into the detailed description of these above-mentioned steps
Before you Get Started
- Sign up for a MirrorFly account.
- Install the following dependencies
- Node – 14.20.0
- npm – 6.14.10
- react-native – 0.69.12
- Install the npm package dependencies
{
"@react-native-async-storage/async-storage": "^1.17.7",//Any version
"react-native-get-random-values": "1.7.1",//must use version >=1.7.1
"react-native-document-picker" :"8.1.1",// must use version >=8.1.1
'realm': "^10.8.0",
'react-native-fs': "^2.18.0",
'@react-native-community/netinfo': "^8.0.0",
'moment': "2.29.4",
'rn-fetch-blob': "^0.12.0",
'react-native-compressor': "1.6.1",
'react-native-convert-ph-asset': "^1.0.3",
'react-native-mov-to-mp4' :"^0.2.2"
}
Step 1: Integrate Chat SDK
- Check for the latest update of your packages.json files.
- If your iOS app is having issues, check if there are any duplicated items in your package files.
- Go to the dist folder and copy all the extracted files.
- Make a new folder within your project and then move all the copied files into that folder.
- Create a file called “SDK.js” to export the SDK objects. You can use the provided code snippets to import the SDKs.
import './index';
const SDK = window.SDK;
export default SDK;
Step 2: Initialize The Chat SDK
To initialize the Chat SDK, you’ll need specific data that reflects changes in the app’s connection status. Here’s how to do it:
- First, place the license key in the “licensekey” parameter.
- Then, use the method provided below to pass this data to the SDK for further processing
const initializeObj = {
apiBaseUrl: `API_URL`,
licenseKey: `LICENSE_KEY`,
isTrialLicenseKey: `TRIAL_MODE`,
callbackListeners: {},
};
await SDK.initializeSDK(initializeObj);
Sandbox Details
function connectionListener(response) {
if (response.status === "CONNECTED") {
console.log("Connection Established");
} else if (response.status === "DISCONNECTED") {
console.log("Disconnected");
}
}
const initializeObj = {
apiBaseUrl: "https://api-preprod-sandbox.mirrorfly.com/api/v1",
licenseKey: "XXXXXXXXXXXXXXXXX",
isTrialLicenseKey: true,
callbackListeners: {
connectionListener
},
};
await SDK.initializeSDK(initializeObj);
Example Response
{
"statusCode": 200,
"message": "Success"
}
Step 3: Register A New User
Here’s how to register a new user:
- Utilize the method provided below to register a new user.
- After registering, you’ll receive a username and password. You can use these credentials to connect to the server using the “connect” method.
await SDK.register(`USER_IDENTIFIER`);
Sample Response
{
statusCode: 200,
message: "Success",
data: {
username: "123456789",
password: "987654321"
}
}
Step 4: Connect To MirrorFly Server
Follow the below steps to connect to the MirrorFly server:
- Use the credentials you obtained during registration to establish a connection with the server.
- After successfully creating the connection, you’ll either receive an approval message with a ‘statusCode of 200’ or an error if there’s an issue.
- You can also monitor the connection status through the connectionListener callback function.
- If there’s an error during the connection process, you’ll receive an error message through a callback.
await SDK.connect(`USERNAME`, `PASSWORD`);
Sample Response
{
message: "Login Success",
statusCode: 200
}
Step 5: Send A Message
To send a message to another user, simply use the following method:
await SDK.sendTextMessage(`TO_USER_JID`, `MESSAGE_BODY`,`MESSAGE_ID`,`REPLY_TO`);
Sample Response
{
"message": "",// String - Success/Error Message
"statusCode": "" // Number - status code
}
Step 6: Receive A Message
To receive a message from another user, you should set up the “messageListener” function. This function gets activated every time you receive a new message or a related event in one-to-one or group chat. Here’s how to initialize the SDK and add this callback method during the process.
function messageListener(response) {
console.log("Message Listener", response);
}
Once the entire process of registration and sync gets completed, you can refer to adding 1:1 and group chat features.
Register
To begin with, there is a need to create a new user account
If you get the autoLogin as true, then this will enable the user to log in with XMPP after successful registration.
Note – If the sandbox mode is set to true, then the users will register into the sandbox mode at the startup itself. |
await SDK.register(`USER_IDENTIFIER`, `AUTO_LOGIN`);
Sample Responses -
If autoLogin is true
{
statusCode: 200,
message: "Login Success"
}
if autoLogin is false
{
statusCode: 200,
message: "Success",
data: {
username: "123456789",
password: "987654321"
}
}
Now use the credentials that have been received from the Register response, log in using SDK
await SDK.login(`USERNAME`, `PASSWORD`);
Check for further Request and Responses
Example Request
Image as a URL
await SDK.setUserProfile(
"Name",
"https://domain/path/image.png",
"status",
"1111111111",
"email@domain",
);
Image as a FILE
await SDK.setUserProfile("Name", FILE, "status", "1111111111", "email@domain");
When you want to sync the contacts with the License Key, use can use the Sync Contact Method
await SDK.syncContacts(`USER_IDENTIFIER`);
Once the entire process of registration and sync gets completed, you can refer to other profiles using one-to-one chat, and group chat methods.
Well, after having better clarity with the importance of implementation of React Native in your chat app. Let’s get some ideas about the market launch strategy.
Recommended Reading
8 High-end Benefits of A React Native Chat App
- Highly Compatible across the Platforms – specifies that the application that has been coded for Android can also be shared for iOS, which indeed saves time as well as cost.
- Robust Community – this provides access to user support and many other user-created tools.
- Cost Effective and Time Saving – as you can create a single app and use it for both iOS as well as Android.
- Top Reloading Feature – works across all mobile platforms with ease.
- OTA (Over The Air) – an innovative update that enables developers to let their users create and administer recent updates.
- Highly Flexible – This allows any number of developers to work on the app project at once without any interruption enabling them to add any number of features.
- Open Source Framework – this attribute provides the developers with easy accessibility to migrate from one platform to another.
Key Points to Be Considered Before Launching a React Native Chat App!
The main target of every application is to reach the market, but this is not an easy journey. Let’s have a look at some of the key points that every React native chat app has to be considered before making market launch
- Chat Performance & Reliability: Once the chat app using react native SDK is developed, it is time for it to be tested for its performance and reliability. The performance issues will be detected upon real-time experience with perceptible latency, undelivered messages, or any app crash during the course.
- Privacy & Security Protocols: Legal issues like Terms of use and privacy policy are considered to be major issues as they need to be very accurate and must be prepared under the surveillance of a lawyer before launching through the play store.
However, there are many other factors too but above mentioned are some major points for better understanding. Now, let’s see with MirrorFly is the one for this entire React native chat app development.
Why Choose MirrorFly SDK to Build Your React Native Chat App?
When it comes to real-time communication API & SDK providers, you can find several out there – among which, choosing the best one is a big deal. But still, you can have them identified with certain key points like market experience, features, pricing, and so on.
Let’s talk about such one – The “MirrorFly,” one among the top chat API and SDK provider who have marked their market existence for more than 10 year with over 40+ worldwide satisfied customers. Well, let’s get to know more about in detail with some of the highlights
- Real-Time Communication Platform – MirroFly works with multiple modes of communication that involve messaging, voice and video. It is a highly secure solution with over 150+ chat features that fit into the demands of all kinds of businesses.
- High-Scalability – Enables to scale up to 1 billion + concurrent users around the world anytime with high-end security protocols of enterprise-grade.
- High-end Security – It provides end-to-end security over the conversations among the users globally across multiple channels with SSL security, AES 256 encryption, multi-layer protection, etc.
- Hosting Option – You can avail of both on-premises and on-cloud hosting infrastructure, wherein you can have your data stored in your cloud or else in our cloud/premises as per your business requirements with guaranteed security.
- 300+ Proficient Developers – Highly professional with perfection when it comes to an app’s delivery. MirrorFly ensures you have on-time delivery on your projects with 24/7 developers’ assistance till deployment.
- White Label Solution – MirrorFly offers ready-to-use react native chat APIs and SDKs that support you to build your white label chat app with your company’s name, logo, color and custom features. This can be easily published into any play store like Google Play, etc.
- Pricing Model – Since it is available with both SaaS and Saap-based models, you can find both pricing models here i.e., Self-managed chat solution (one-time license cost) and Cloud (pay-as-you-go) pricing model as per your business requirement.
Final Words!
I hope this chat app tutorial would have given you a better idea as to how we built a multi-tenant React Native chat app using the React Native SDKs and component library and of course, with details on how to integrate them into any pre-build device with simple changes in codes.
Well, after all this session if you feel this tutorial is an end for searching for the best mobile app development company, then feel free to contact us for further guidance. By the way, you can also check our other tutorials, for Android and iOS for better exposure.
All the Best towards the Journey to Your Chat App. Thank You!
Get Started with MirrorFly’s React Native Chat API
Drive 1+ billions of conversations on your apps with highly secure 250+ real-time Communication Features.
Contact Sales200+ Happy Clients
Topic-based Chat
Multi-tenancy Support
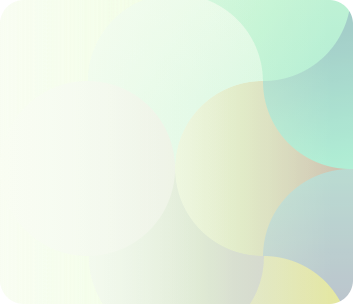
Frequently Asked Questions (FAQs)
Does WhatsApp use React Native?
No, WhatsApp doesn’t use React Native in its UI components. WhatsApp is being developed natively using platform-specific applications such as Objective-C and Swift for iOS and Kotlin and Java for Android. But as technology grows, new frameworks can be adopted by brands depending on their project requirements and programming features.
How do I make a native React chat app for free?
To build your own React Native chat app for free, you can hire a team of developers or follow the steps to create your own React Native chat app for free. But there are other options too; you can go through a list of free chat SDK providers like MirrorFly, Getstream, Cometchat, etc., and easily integrate them into your preferred platform.
How do I create a group chat in React Native?
To create a group chat in React Native, you can follow these simple steps:
Step 1: Set up the React Native project.
Step 2: Design the user interface
Step 3: Implement navigation
Step 4: Connect to the backend
Step 5: Fetch group chat data.
Step 6: Create or join a group chat.
Step 7: Display or send group chat messages.
Can I use Firebase with React Native?
Yes, you can use Firebase with React Native. Firebase’s wide portfolio of cloud-based services can be easily integrated into your React Native applications. It offers features such as a real-time database, authentication, cloud storage, messaging, and more, which can simplify the development process.
Is React a native open-source framework?
Yes, React Native is an open-source framework initially developed and released by Facebook in 2015. As an open-source framework, React Native allows developers to migrate from one platform to another. The source code of React Native is hosted on Github, which allows developers the freedom to use, modify, and distribute the code for personal and commercial needs.
Which famous brands use React Native?
Facebook, Instagram, Airbnb, Tesla, Bloomberg, Walmart, etc. are some of the popular brands that have adopted the React Native framework for their mobile applications UI components. The adoption of React Native by these popular brands proves the framework’s creativity, performance, and ability to deliver cross-platform mobile experiences proficiently.
Related Articles
What is in-app messaging React Native?
In the context of chatting between users within an app, In-App Messaging in React Native refers to the feature that allows users to engage in real-time conversations or chats with each other while using the mobile application. This enables users to exchange messages and communicate with one another directly within the app, enhancing their in-app experience.
What is the backend language of React Native?
React Native itself does not have a specific backend language. It is primarily a framework for building mobile applications, and it uses JavaScript to develop the front-end of the app. For the backend of a React Native app, you have the flexibility to use various technologies. One popular choice is Node.js, which is a JavaScript runtime that enables the development of server-side applications. However, you can use other backend languages and frameworks like Python, Ruby on Rails, Java, or PHP, depending on your project requirements and preferences.
Which app is faster Flutter or React Native?
Flutter is generally considered to be faster than React Native because it doesn’t rely on JavaScript bridging, which can introduce some performance overhead in React Native. However, it’s important to note that Flutter apps can have a larger file size, which may be a concern for developers. So, in terms of raw performance, Flutter is often faster, but you need to consider other factors like file size when making a choice between the two frameworks.
What are the different types of notifications in React Native?
React Native supports various types of notifications, including remote, local, interactive, and silent notifications, covering all aspects of push notifications for your app. It also fully supports native iOS notification features.
hello we are building an react native chat app and need a chat room for 30 people with mutplie room option api for mobile . we need also streaming to call people one to one .please email
Hi, we would like to know the terms of use of your functionality and the possibility of customizing tariff plans to suit our needs. We are a company that develops a classified service where there is a chat functionality between users, and we wanted to find out if your service is suitable for us
Would like to inquire about your prices for building a chat massaging app using react native app with features like what’s app for South African environment…
Hi, our sites has more than 100,000 users visit last month (400k, est). However, there are only 30k active users. Most of the visits are just new users that might not end up registe. Still, we want to expose the chat feature to them. (They can only see chat message/history — cant send message) We want to discuss about how MirrorFly calculate MAU, price.