Build Group Video Chat App using Node.js + WebRTC
Looking to Build Peer-to-Peer Video Chat App with Node.js?
In this article, we will discuss how to build a live video chat app WebRTC technology and Node.js
If you are acquainted with any sort of real-time meetings that use the internet to communicate – then chances are you have used WebRTC. Google Meet, Discord, Facebook Messenger, GoToMeeting, Amazon Chime, etc., are some of the top video chat app that make use of WebRTC js.
Through this article, you as a developer can learn how to build a web application or chat apps with video calls. This is basically a guide that will help developers understand how to create a live video call app that allows a user to broadcast their video and voice in their room. This room will be available for other users, called viewers, giving them an option to join as the approval of the host of the meeting. The viewers will be able to interact with the broadcaster via a chat functionality that will deliver messages to the entire chat room.
To create chat application, you will use Node.JS for the server framework. Web Sockets will handle the communication between peers in each room and WebRTC will manage the broadcasting of the video and voice. But before we get to that, let’s understand what WebRTC is and define thoroughly what it means.
Table of Contents
What is WebRTC?
WebRTC is an open framework for managing real-time communications. WebRTC stands for Web Real-Time Communication and is basically a technology that allows web applications and sites to capture and / or stream audio and/ or videos. It also enables users to exchange arbitrary data between browsers without requiring an intermediary. In simple words, it helps in transmitting video, voice, or any data between peers.
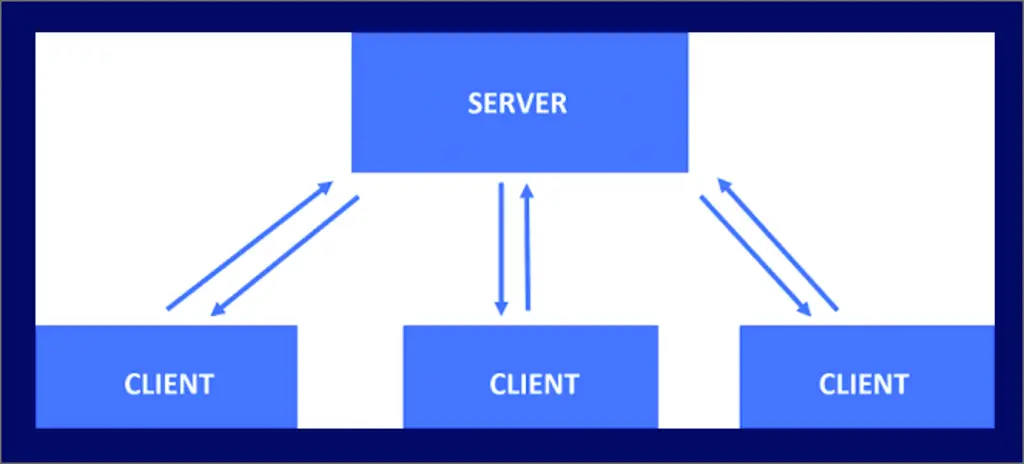
WebRTC is an HTML5 specification that you as a WebRTC developer can use to add real time media communications directly between browser and devices. WebRTC offers voice and video communication to work within a web page and eliminates additional plugins. It has been extensively supported by Google, Apple, Microsoft, Mozilla, among others. Moreover, as it is free and comes as an open-source project embedded in the browsers – it is easier to adopt and amend as per requirements.
This has created a vibrant and dynamic ecosystem around WebRTC of a variety of open-source projects and frameworks as well as commercial offerings from companies that help you to build your products based on WebRTC alone.
7 Steps: Make a Group Video Chat App with WebRTC & Node.js
Step 1: Quick Note on Testing and Debugging
If you try to open file://your-webrtc-project in your browser, you will likely run into Cross-Origin Resource Sharing (CORS) errors since the browser will block your requests to use video and microphone features.
To test your code, you have a few options. You can upload your files to a web server, like Github Pages if you prefer. However, to keep development local, it is recommended you set up a simple server using Python. To do this, open your terminal and change directories into your current project and depending on your version of Python, run one of the following modules.
For example, if we run Python2.7 and the command we can use is python -m SimpleHTTPServer 8001. Go to http://localhost:8001/index.html to debug my app. Try making an index.html with anything in it and serve it on localhost before continuing.
Step 2: The HTML5 Backbone
For the sake of the demo, let’s keep the HTML short and simple. First, we need a div to house our videos. Then, all we really need to start off with is a login field so you can specify your name and a call field so you can dial someone.
<div id=”vid-box”></div>
<form name=”loginForm” id=”login” action=”#” onsubmit=”return login(this);”>
<input type=”text” name=”username” id=”username” placeholder=”Pick a username!” />
<input type=”submit” name=”login_submit” value=”Log In”>
</form>
<form name=”callForm” id=”call” action=”#” onsubmit=”return makeCall(this);”>
<input type=”text” name=”number” placeholder=”Enter user to dial!” />
<input type=”submit” value=”Call”/>
</form>
This should leave you with an elaborate, well styled HTML backbone that looks something like the image header to this step.
Step 3: The JavaScript Imports
There are three libraries that you will need to include to make WebRTC operations much easier:
Include jQuery to make modifying DOM elements a breeze.
Include the JavaScript SDK to facilitate the WebRTC signaling (more on signaling at the bottom of this blog post).
Include the WebRTC SDK to make placing phone calls as simple as calling the dial(number)function.
Now we’re ready to write our calling functions for login and makeCall.
Step 4: Preparing to Receive Calls
In order to start facilitating video calls, you will need a publish and subscribe key. To get your sub keys, you will first need to sign up for a MirrorFly account. Once you sign up, you can find your unique MirrorFly keys in the MirrorFly Developer Dashboard. The free Sandbox tier should give you all the bandwidth you need to build and test your WebRTC application.
First, let’s use JavaScript to find our video holder, where other caller’s faces will go.
var video_out = document.getElementById(“vid-box”);
Next, we’ll implement the login function. This function will set up the phone using the username they provided as a UUID.
var video_out = document.getElementById(“vid-box”);
Next, we’ll implement the login function. This function will set up the phone using the username they provided as a UUID.
function login(form) {
var phone = window.phone = PHONE({
number : form.username.value || “Anonymous”, // listen on username line else Anonymous
publish_key : ‘your_pub_key’,
subscribe_key : ‘your_sub_key’,
});
phone.ready(function(){ form.username.style.background=”#55ff5b”; });
phone.receive(function(session){
session.connected(function(session) { video_out.appendChild(session.video); });
session.ended(function(session) { video_out.innerHTML=”; });
});
return false; // So the form does not submit.
}
Step 5: Making Calls
We now have our WebRTC video app ready to receive a call, so it is time to create a makeCall function.
if (!window.phone) alert(“Login First!”);
else phone.dial(form.number.value);
return false;
}
If window.phone is undefined, we cannot place a call. This will happen if the user did not log in first. If it exists, we use the phone.dial function which takes a number and an optional list of servers to place a call.
And that is it! You now have a simple WebRTC video chat app. Fire up your python server and go test your app on localhost!
In our next two parts, we walk through how to add a number of additional features to your video chat app, including make/end Calls, thumbnail streams, mute calls, pause videos, and group chatting. We’ll also walk through how to create live embeddable streaming content (ie. how to build Periscope with WebRTC!).
Step 6: Why WebRTC Video Call API?
Embedded with audio-video communication, WebRTC enables users to consume very little bandwidth and offers zero latency. This is further supported on all major browsers and mobile devices which makes using WebRTC extremely beneficial.
Step 7: Want to Learn More?
WebRTC is a prevailing technology, will stay around for a long time, and is a great skill to have.
How Does a WebRTC Video Call App Works?
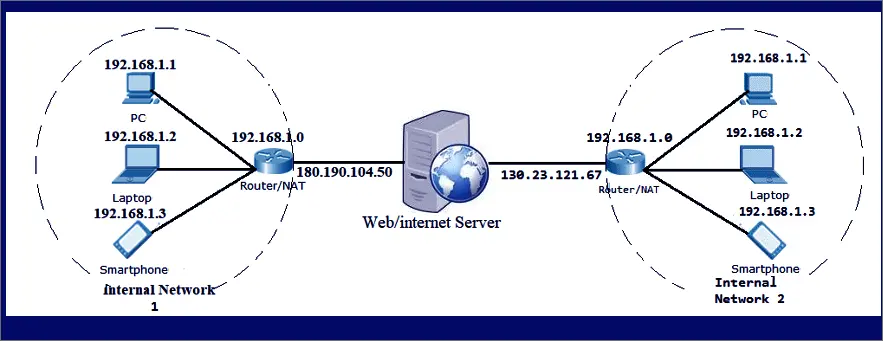
WebRTC works based on codecs. These are algorithms that are used to compress and decompress audio, video, and data. There are different codecs you can use in WebRTC that can send audio and video files with low latency in mind. WebRTC uses known VoIP techniques to get media processed and sent through the network, and this is all done over SRTP – the secure and encrypted version of RTP.
After signaling – Use ICE to cope with NATs and firewalls
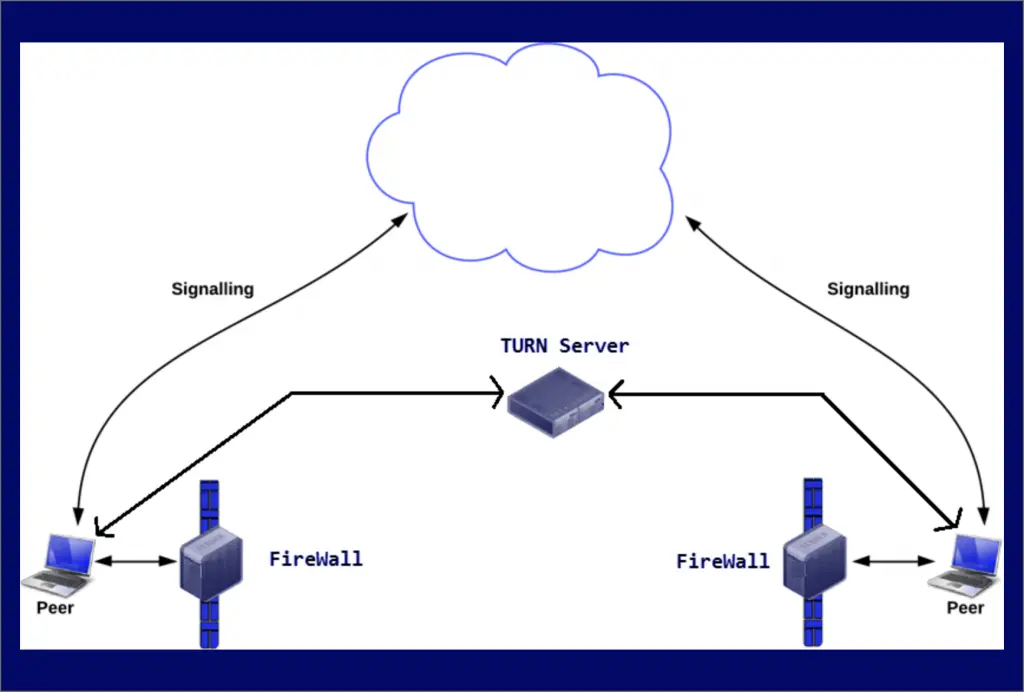
WebRTC apps can use the ICE framework to overcome the complexities of real-world networking. After signaling an app must pass ICE server URLs to RTCPeerConnection. ICE tries to find the best path to connect with peers. It tries all possibilities in parallel and chooses the most efficient option that works. ICE first tries to make a connection using the host address obtained from a device’s operating system and network card. If that fails (which it will for devices behind NATs), ICE obtains an external address using a STUN server and, if that fails, traffic is routed through a TURN relay server.
function login(form)
{
var phone = window.phone = PHONE
({ number
: form.username.value || “Anonymous”,
// listen on username line else Anonymous
publish_key : ‘your_pub_key’,
subscribe_key : ‘your_sub_key’, });
phone.ready(function()
{
form.username.style.background=”#55ff5b”;
});
phone.receive(function(session)
{
session.connected(function(session)
{
video_out.appendChild(session.video);
});
session.ended(function(session)
{
video_out.innerHTML=”;
});
}); return false;
// So the form does not submit. }
Recommended Reading
Summing Up!
We hope that this article has helped you to gain insight into the concepts and connections with regards to WebRTC video call P2P communication. And, how to efficiently build a peer-to-peer video chat app with Node.js.
Besides, if you are looking to develop in-app chats with 100% customizable video calling features, check out this self-hosted solution.
Get Started with MirrorFly’s Secure WebRTC Video Call!
Drive 1+ billions of conversations on your apps with highly secure 250+ real-time Communication Features.
Contact Sales200+ Happy Clients
Topic-based Chat
Multi-tenancy Support
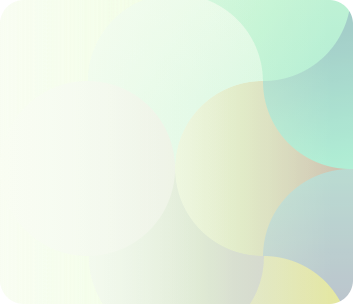
Further Article
- What is a Video SDK? – An Ultimate Guide [2024]
- 10 Best Video Calling APIs and Conferencing SDKs in 2024
- How to Embed Video Calls to Your Website in Easy Steps?
- Build vs Buy: A Guide To Developers On Video and WebRTC
- Live Video Call: How Can It Benefit Your Business In 2024?
- Top 10 WebRTC Video Call Solutions
Frequently Asked Questions (FAQs)
What is WebRTC node JS?
WebRTC node JS is a Native Addon of Node.JS which is used to bind the WebRTC M87. It mainly aims on spec-compliance which is tested using the W3C’s web-platform-tests project. This also includes the number of nonstandard APIs for testing.
How does WebRTC peer-to-peer work?
Generally, in WebRTC the communication takes place as peer-to-peer or across the browser, instead of client-server connection. Here, the connection is equally distributed between the two communicating agents using their transport addresses.
Let’s have a look in detail,
- First WebRTC send across the data like video, audio and other files by making use of NAT traversal mechanisms for browsers to reach each other.
- Then the P2P needs to be go through a relay server (TURN).
- Later WebRTC needs to think about signaling and media which is totally different from each other,
– It allows the peer-to-peer communication with little intervention of a server that’s mainly used for signaling alone.
– Moreover, it is possible to hold video calls with multiple participants using peer-to-peer communication. Here the media server helps to reduce the number of streams that a client needs to send or receive.
Server’s that are been used in a WebRTC includes,
- Signaling server
- STUN/TURN servers
- Media server
What is an example of a peer-to-peer network?
Bluetooth that’s used in Android devices is one of the most common examples of a peer-to-peer network with which you can share files over the internet. Even the online gaming platforms make use of P2P to download the games among the users. This includes Starcraft II, World of Warcraft, Blizzard Entertainment, and more.
What is the most common type of P2P network?
The most common type of structured P2P network is Bittorrent network which implements the distributed hash table (DHT), where it connects the network of computers to each other to share data and electronic files.
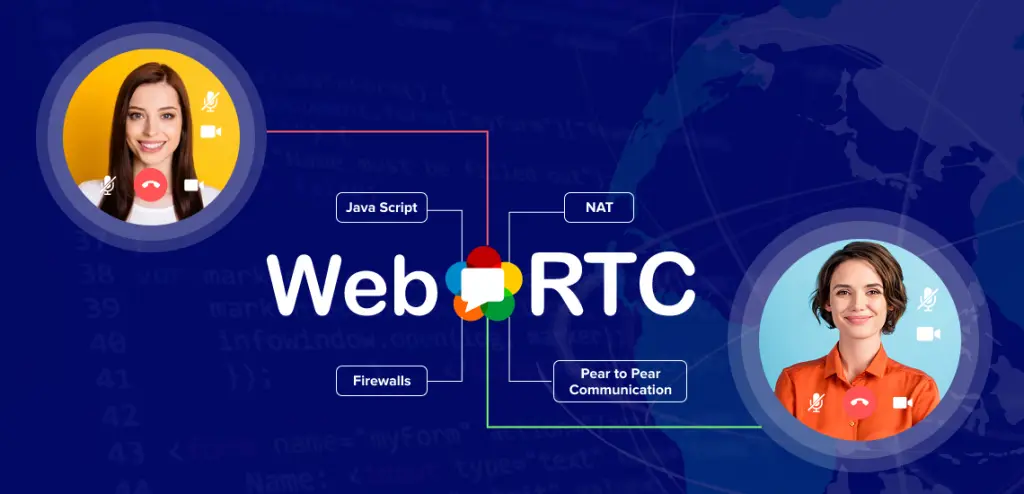
Looking to Build Peer-to-Peer Video Chat App with Node.js?
In this article, we will discuss how to build a live video chat app WebRTC technology and Node.js
If you are acquainted with any sort of real-time meetings that use the internet to communicate – then chances are you have used WebRTC. Google Meet, Discord, Facebook Messenger, GoToMeeting, Amazon Chime, etc., are some of the top video chat app that make use of WebRTC js.
Through this article, you as a developer can learn how to build a web application or chat apps with video calls. This is basically a guide that will help developers understand how to create a live video call app that allows a user to broadcast their video and voice in their room. This room will be available for other users, called viewers, giving them an option to join as the approval of the host of the meeting. The viewers will be able to interact with the broadcaster via a chat functionality that will deliver messages to the entire chat room.
To create chat application, you will use Node.JS for the server framework. Web Sockets will handle the communication between peers in each room and WebRTC will manage the broadcasting of the video and voice. But before we get to that, let’s understand what WebRTC is and define thoroughly what it means.
Table of Contents
What is WebRTC?
WebRTC is an open framework for managing real-time communications. WebRTC stands for Web Real-Time Communication and is basically a technology that allows web applications and sites to capture and / or stream audio and/ or videos. It also enables users to exchange arbitrary data between browsers without requiring an intermediary. In simple words, it helps in transmitting video, voice, or any data between peers.
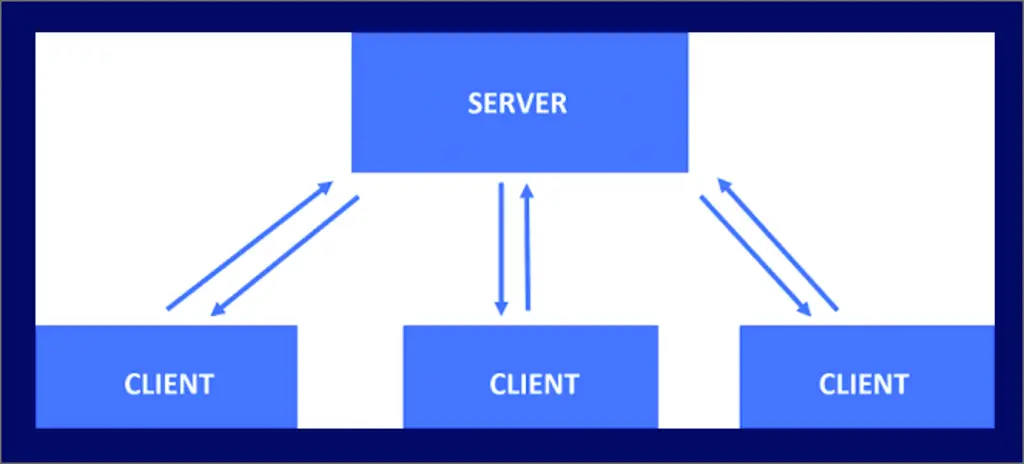
WebRTC is an HTML5 specification that you as a WebRTC developer can use to add real time media communications directly between browser and devices. WebRTC offers voice and video communication to work within a web page and eliminates additional plugins. It has been extensively supported by Google, Apple, Microsoft, Mozilla, among others. Moreover, as it is free and comes as an open-source project embedded in the browsers – it is easier to adopt and amend as per requirements.
This has created a vibrant and dynamic ecosystem around WebRTC of a variety of open-source projects and frameworks as well as commercial offerings from companies that help you to build your products based on WebRTC alone.
7 Steps: Make a Group Video Chat App with WebRTC & Node.js
Step 1: Quick Note on Testing and Debugging
If you try to open file://your-webrtc-project in your browser, you will likely run into Cross-Origin Resource Sharing (CORS) errors since the browser will block your requests to use video and microphone features.
To test your code, you have a few options. You can upload your files to a web server, like Github Pages if you prefer. However, to keep development local, it is recommended you set up a simple server using Python. To do this, open your terminal and change directories into your current project and depending on your version of Python, run one of the following modules.
For example, if we run Python2.7 and the command we can use is python -m SimpleHTTPServer 8001. Go to http://localhost:8001/index.html to debug my app. Try making an index.html with anything in it and serve it on localhost before continuing.
Step 2: The HTML5 Backbone
For the sake of the demo, let’s keep the HTML short and simple. First, we need a div to house our videos. Then, all we really need to start off with is a login field so you can specify your name and a call field so you can dial someone.
<div id=”vid-box”></div>
<form name=”loginForm” id=”login” action=”#” onsubmit=”return login(this);”>
<input type=”text” name=”username” id=”username” placeholder=”Pick a username!” />
<input type=”submit” name=”login_submit” value=”Log In”>
</form>
<form name=”callForm” id=”call” action=”#” onsubmit=”return makeCall(this);”>
<input type=”text” name=”number” placeholder=”Enter user to dial!” />
<input type=”submit” value=”Call”/>
</form>
This should leave you with an elaborate, well styled HTML backbone that looks something like the image header to this step.
Step 3: The JavaScript Imports
There are three libraries that you will need to include to make WebRTC operations much easier:
Include jQuery to make modifying DOM elements a breeze.
Include the JavaScript SDK to facilitate the WebRTC signaling (more on signaling at the bottom of this blog post).
Include the WebRTC SDK to make placing phone calls as simple as calling the dial(number)function.
Now we’re ready to write our calling functions for login and makeCall.
Step 4: Preparing to Receive Calls
In order to start facilitating video calls, you will need a publish and subscribe key. To get your sub keys, you will first need to sign up for a MirrorFly account. Once you sign up, you can find your unique MirrorFly keys in the MirrorFly Developer Dashboard. The free Sandbox tier should give you all the bandwidth you need to build and test your WebRTC application.
First, let’s use JavaScript to find our video holder, where other caller’s faces will go.
var video_out = document.getElementById(“vid-box”);
Next, we’ll implement the login function. This function will set up the phone using the username they provided as a UUID.
var video_out = document.getElementById(“vid-box”);
Next, we’ll implement the login function. This function will set up the phone using the username they provided as a UUID.
function login(form) {
var phone = window.phone = PHONE({
number : form.username.value || “Anonymous”, // listen on username line else Anonymous
publish_key : ‘your_pub_key’,
subscribe_key : ‘your_sub_key’,
});
phone.ready(function(){ form.username.style.background=”#55ff5b”; });
phone.receive(function(session){
session.connected(function(session) { video_out.appendChild(session.video); });
session.ended(function(session) { video_out.innerHTML=”; });
});
return false; // So the form does not submit.
}
Step 5: Making Calls
We now have our WebRTC video app ready to receive a call, so it is time to create a makeCall function.
if (!window.phone) alert(“Login First!”);
else phone.dial(form.number.value);
return false;
}
If window.phone is undefined, we cannot place a call. This will happen if the user did not log in first. If it exists, we use the phone.dial function which takes a number and an optional list of servers to place a call.
And that is it! You now have a simple WebRTC video chat app. Fire up your python server and go test your app on localhost!
In our next two parts, we walk through how to add a number of additional features to your video chat app, including make/end Calls, thumbnail streams, mute calls, pause videos, and group chatting. We’ll also walk through how to create live embeddable streaming content (ie. how to build Periscope with WebRTC!).
Step 6: Why WebRTC Video Call API?
Embedded with audio-video communication, WebRTC enables users to consume very little bandwidth and offers zero latency. This is further supported on all major browsers and mobile devices which makes using WebRTC extremely beneficial.
Step 7: Want to Learn More?
WebRTC is a prevailing technology, will stay around for a long time, and is a great skill to have.
How Does a WebRTC Video Call App Works?
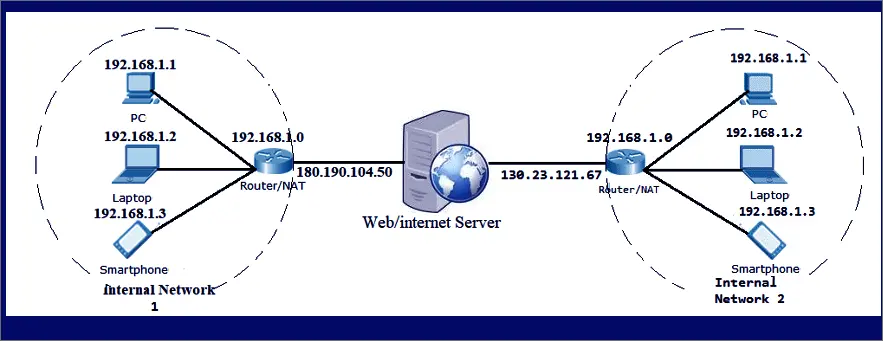
WebRTC works based on codecs. These are algorithms that are used to compress and decompress audio, video, and data. There are different codecs you can use in WebRTC that can send audio and video files with low latency in mind. WebRTC uses known VoIP techniques to get media processed and sent through the network, and this is all done over SRTP – the secure and encrypted version of RTP.
After signaling – Use ICE to cope with NATs and firewalls
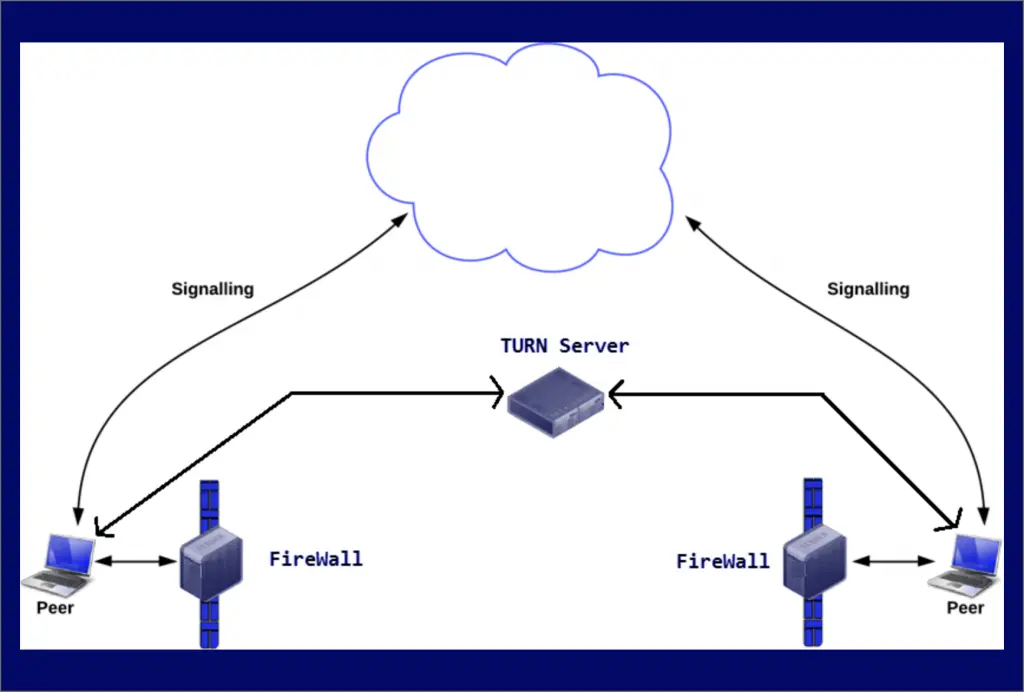
WebRTC apps can use the ICE framework to overcome the complexities of real-world networking. After signaling an app must pass ICE server URLs to RTCPeerConnection. ICE tries to find the best path to connect with peers. It tries all possibilities in parallel and chooses the most efficient option that works. ICE first tries to make a connection using the host address obtained from a device’s operating system and network card. If that fails (which it will for devices behind NATs), ICE obtains an external address using a STUN server and, if that fails, traffic is routed through a TURN relay server.
function login(form)
{
var phone = window.phone = PHONE
({ number
: form.username.value || “Anonymous”,
// listen on username line else Anonymous
publish_key : ‘your_pub_key’,
subscribe_key : ‘your_sub_key’, });
phone.ready(function()
{
form.username.style.background=”#55ff5b”;
});
phone.receive(function(session)
{
session.connected(function(session)
{
video_out.appendChild(session.video);
});
session.ended(function(session)
{
video_out.innerHTML=”;
});
}); return false;
// So the form does not submit. }
Recommended Reading
Summing Up!
We hope that this article has helped you to gain insight into the concepts and connections with regards to WebRTC video call P2P communication. And, how to efficiently build a peer-to-peer video chat app with Node.js.
Besides, if you are looking to develop in-app chats with 100% customizable video calling features, check out this self-hosted solution.
Get Started with MirrorFly’s Secure WebRTC Video Call!
Drive 1+ billions of conversations on your apps with highly secure 250+ real-time Communication Features.
Contact Sales200+ Happy Clients
Topic-based Chat
Multi-tenancy Support
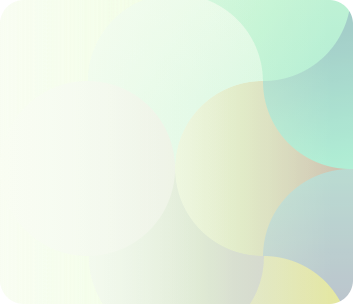
Further Article
- What is a Video SDK? – An Ultimate Guide [2024]
- 10 Best Video Calling APIs and Conferencing SDKs in 2024
- How to Embed Video Calls to Your Website in Easy Steps?
- Build vs Buy: A Guide To Developers On Video and WebRTC
- Live Video Call: How Can It Benefit Your Business In 2024?
- Top 10 WebRTC Video Call Solutions
Frequently Asked Questions (FAQs)
What is WebRTC node JS?
WebRTC node JS is a Native Addon of Node.JS which is used to bind the WebRTC M87. It mainly aims on spec-compliance which is tested using the W3C’s web-platform-tests project. This also includes the number of nonstandard APIs for testing.
How does WebRTC peer-to-peer work?
Generally, in WebRTC the communication takes place as peer-to-peer or across the browser, instead of client-server connection. Here, the connection is equally distributed between the two communicating agents using their transport addresses.
Let’s have a look in detail,
- First WebRTC send across the data like video, audio and other files by making use of NAT traversal mechanisms for browsers to reach each other.
- Then the P2P needs to be go through a relay server (TURN).
- Later WebRTC needs to think about signaling and media which is totally different from each other,
– It allows the peer-to-peer communication with little intervention of a server that’s mainly used for signaling alone.
– Moreover, it is possible to hold video calls with multiple participants using peer-to-peer communication. Here the media server helps to reduce the number of streams that a client needs to send or receive.
Server’s that are been used in a WebRTC includes,
- Signaling server
- STUN/TURN servers
- Media server
What is an example of a peer-to-peer network?
Bluetooth that’s used in Android devices is one of the most common examples of a peer-to-peer network with which you can share files over the internet. Even the online gaming platforms make use of P2P to download the games among the users. This includes Starcraft II, World of Warcraft, Blizzard Entertainment, and more.
What is the most common type of P2P network?
The most common type of structured P2P network is Bittorrent network which implements the distributed hash table (DHT), where it connects the network of computers to each other to share data and electronic files.
Hi Jay!
Yes, ApphiTect supports one-to-one video calls using WebRTC, group messages and up to 1000+ in-app communication features with dedicated developer support for the end-to-end integration process. Contact our sales team to learn more details.
Hi, my team and I are currently working on two apps and we would like to use your video call solution. How does the pricing work? We are pre-traction so our initial usage would be very small.
Hi Vadim!
We really appreciate your comment. Based on your requirements, ApphiTect offers both SaaS and self-hosted solutions for building a WebRTC video and voice chat application. You can contact the team for a free consultation and pricing.
Hello we are a start up e-learning company we have not launched yet but we are interested in some of you services. We would like to make use of your 1-to-1 video chat feature, and your group message feature
Hi Team, I am looking for an webrtc video chat app that can provide voice, video and messaging features and I am sure that your sdk can provide me with all the functionalities needded. Can you please give me your pricing breakdown please? Kind Regards
Hi Ristel!
Thanks for your comment. ApphiTect Chat SDK will be the best choice for WebRTC group video and private video calls. Since this is a self-hosted messaging solution, you can customize, add, remove and change all the functionalities you require.
ApphiTect has both SaaS and SaaP pricing models. Contact our team for more information.
Hi, we are a fintech company located in Singapore and Indonesia, we are looking to build peer-to-peer video chat app. Would be great if we can arrange time for a discussion. Thanks.
Hi Maria,
We’re glad to discuss your requirement for a WebRTC peer-to-peer video call for Fintech. Please initiate a Request Demo; our team will contact you.
We need to integrate video/voice peer-to-peer chat on our existing app. what could be the costs? Is your video SDK fit for my business requirements
Hi Ahamed! Of course, ApphiTect WebRTC Video SDK supports all business requirements across industries and use cases. It also supports all tech stacks, programming languages, and browsers. You can also hire a dedicated team for the integration process. Contact for more details.
I want to start my own peer to peer video Chatting App with webrtc and Java